Contents:
.outerHeight( [includeMargin ] )Returns: Number
Description: Get the current computed outer height (including padding, border, and optionally margin) for the first element in the set of matched elements.
-
version added: 1.2.6.outerHeight( [includeMargin ] )
-
includeMargin (default:
false
)Type: BooleanA Boolean indicating whether to include the element's margin in the calculation.
-
Returns the height of the element, including top and bottom padding, border, and optionally margin, in pixels. If called on an empty set of elements, returns undefined
(null
before jQuery 3.0).
This method is not applicable to window
and document
objects; for these, use .height()
instead. Although .outerHeight()
can be used on table elements, it may give unexpected results on tables using the border-collapse: collapse
CSS property.
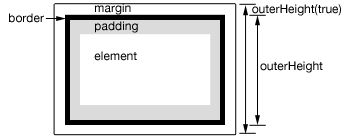
Additional Notes:
-
The number returned by dimensions-related APIs, including
.outerHeight()
, may be fractional in some cases. Code should not assume it is an integer. Also, dimensions may be incorrect when the page is zoomed by the user; browsers do not expose an API to detect this condition. -
The value reported by
.outerHeight()
is not guaranteed to be accurate when the element or its parent is hidden. To get an accurate value, ensure the element is visible before using.outerHeight()
. jQuery will attempt to temporarily show and then re-hide an element in order to measure its dimensions, but this is unreliable and (even when accurate) can significantly impact page performance. This show-and-rehide measurement feature may be removed in a future version of jQuery.
Example:
Get the outerHeight of a paragraph.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
|
|
Demo:
.outerHeight( value [, includeMargin ] )Returns: jQuery
Description: Set the CSS outer height of each element in the set of matched elements.
-
version added: 1.8.outerHeight( value [, includeMargin ] )
-
valueA number representing the number of pixels, or a number along with an optional unit of measure appended (as a string).
-
includeMargin (default:
false
)Type: BooleanA Boolean indicating whether to new value should account for the element's margin.
-
-
version added: 1.8.outerHeight( function )
-
functionA function returning the outer height to set. Receives the index position of the element in the set and the old outer height as arguments. Within the function,
this
refers to the current element in the set.
-
When calling .outerHeight(value)
, the value can be either a string (number and unit) or a number. If only a number is provided for the value, jQuery assumes a pixel unit. If a string is provided, however, any valid CSS measurement may be used (such as 100px
, 50%
, or auto
).
Example:
Change the outer height of each div the first time it is clicked (and change its color).
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
|
|