Contents:
.on( "mouseenter" [, eventData ], handler )Returns: jQuery
Description: Bind an event handler to be fired when the mouse enters an element.
-
version added: 1.7.on( "mouseenter" [, eventData ], handler )
This page describes the mouseenter
event. For the deprecated .mouseenter()
method, see .mouseenter()
.
The mouseenter
JavaScript event is proprietary to Internet Explorer. Because of the event's general utility, jQuery simulates this event so that it can be used regardless of browser. This event is sent to an element when the mouse pointer enters the element. Any HTML element can receive this event.
For example, consider the HTML:
1
2
3
4
5
6
7
8
9
10
|
|
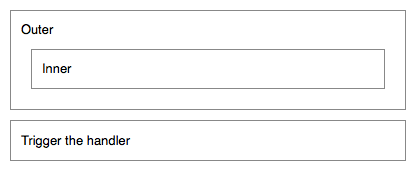
The event handler can be bound to any element:
1
2
3
|
|
Now when the mouse pointer moves over the Outer <div>
, the message is appended to <div id="log">
. You can also trigger the event when another element is clicked:
1
2
3
|
|
After this code executes, clicks on Trigger the handler will also append the message.
The mouseenter
event differs from mouseover
in the way it handles event bubbling. If mouseover
were used in this example, then when the mouse pointer moved over the Inner element, the handler would be triggered. This is usually undesirable behavior. The mouseenter
event, on the other hand, only triggers its handler when the mouse enters the element it is bound to, not a descendant. So in this example, the handler is triggered when the mouse enters the Outer element, but not the Inner element.
Example:
Show texts when mouseenter and mouseout event triggering.
mouseover
fires when the pointer moves into the child element as well, while mouseenter
fires only when the pointer moves into the bound element.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
|
|
Demo:
.trigger( "mouseenter" )Returns: jQuery
Description: Trigger the "mouseenter" event on an element.
-
version added: 1.0.trigger( "mouseenter" )
-
"mouseenter"Type: stringThe string
"mouseenter"
.
-
See the description for .on( "mouseenter", ... )
.