Contents:
.on( "mouseover" [, eventData ], handler )Returns: jQuery
Description: Bind an event handler to the "mouseover" event.
-
version added: 1.7.on( "mouseover" [, eventData ], handler )
This page describes the mouseover
event. For the deprecated .mouseover()
method, see .mouseover()
.
The mouseover
event is sent to an element when the mouse pointer enters the element. Any HTML element can receive this event.
For example, consider the HTML:
1
2
3
4
5
6
7
8
9
10
|
|
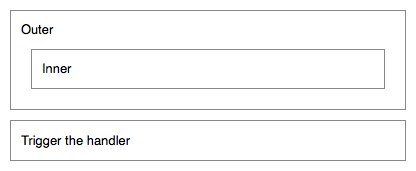
The event handler can be bound to any element:
1
2
3
|
|
Now when the mouse pointer moves over the Outer <div>
, the message is appended to <div id="log">
. We can also trigger the event when another element is clicked:
1
2
3
|
|
After this code executes, clicks on Trigger the handler will also append the message.
This event type can cause many headaches due to event bubbling. For instance, when the mouse pointer moves over the Inner element in this example, a mouseover
event will be sent to that, then trickle up to Outer. This can trigger our bound mouseover
handler at inopportune times. See the discussion for .mouseenter()
for a useful alternative.
Example:
Show the number of times mouseover and mouseenter events are triggered.
mouseover
fires when the pointer moves into the child element as well, while mouseenter
fires only when the pointer moves into the bound element.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
|
|
Demo:
.trigger( "mouseover" )Returns: jQuery
Description: Trigger the "mouseover" event on an element.
-
version added: 1.0.trigger( "mouseover" )
-
"mouseover"Type: stringThe string
"mouseover"
.
-
See the description for .on( "mouseover", ... )
.