Contents:
.on( "click" [, eventData ], handler )Returns: jQuery
Description: Bind an event handler to the "click" event.
-
version added: 1.7.on( "click" [, eventData ], handler )
This page describes the click
event. For the deprecated .click()
method, see .click()
.
The click
event is sent to an element when the mouse pointer is over the element, and the mouse button is pressed and released. Any HTML element can receive this event.
For example, consider the HTML:
1
2
3
4
5
6
|
|
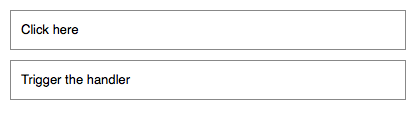
The event handler can be bound to any <div>
:
1
2
3
|
|
Now if we click on this element, the alert is displayed:
Handler for `click` called.
We can also trigger the event when a different element is clicked:
1
2
3
|
|
After this code executes, clicking on Trigger the handler will also alert the message.
The click
event is only triggered after this exact series of events:
- The mouse button is depressed while the pointer is inside the element.
- The mouse button is released while the pointer is inside the element.
This is usually the desired sequence before taking an action. If this is not required, the mousedown
or mouseup
event may be more suitable.
Examples:
Example 1
Hide paragraphs on a page when they are clicked:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
|
|
Demo:
Example 2
Trigger the click event on all the paragraphs on the page:
1
|
|
.trigger( "click" )Returns: jQuery
Description: Trigger the "click" event on an element.
-
version added: 1.0.trigger( "click" )
-
"click"Type: stringThe string
"click"
.
-
See the description for .on( "click", ... )
.